ALL >> Web-Design >> View Article
Navigating The React Native Lifecycle: A Comprehensive Guide To Component Lifecycles
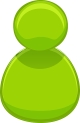
React Native, with its declarative and component-based architecture, provides a powerful framework for building dynamic and responsive mobile applications. Central to the lifecycle of React Native components are a series of methods that define the stages a component goes through, from creation to removal. In this comprehensive guide, we will unravel the intricacies of the React Native component lifecycle, exploring each phase and understanding how to harness its power for effective app development.
Understanding the React Native Component Lifecycle
Initialization Phase:
constructor():
The constructor method is the first to be called when a component is created. It initializes the component's state and binds event handlers. Remember to call super(props) within the constructor to ensure the proper initialization of the parent class.
static getDerivedStateFromProps():
This method is invoked before rendering when new props or state are received. It allows the component to update its internal state based on changes in props.
Mounting Phase:
render():
The render method is ...
... responsible for rendering the component's UI. It should be a pure function, meaning it should not modify the component's state or perform side effects.
componentDidMount():
Invoked immediately after a component is mounted. This is the ideal place to initiate network requests, set up subscriptions, or perform any actions that require access to the DOM.
Updating Phase:
static getDerivedStateFromProps():
As mentioned earlier, this method is also called during the updating phase when new props or state are received.
shouldComponentUpdate():
This method allows the component to control whether it should re-render or not. By default, it returns true, but you can implement custom logic to optimize performance by preventing unnecessary renders.
render():
The render method is called again if shouldComponentUpdate returns true.
getSnapshotBeforeUpdate():
Invoked right before the most recently rendered output is committed to the DOM. It receives the previous props and state, providing an opportunity to capture information for later use.
componentDidUpdate():
Called after the component has been updated in the DOM. It's suitable for performing side effects such as data fetching based on changes.
Unmounting Phase:
componentWillUnmount():
Invoked just before a component is unmounted and destroyed. It's the cleanup phase, where you can cancel network requests, unsubscribe from subscriptions, or perform any necessary cleanup.
React Native Component Lifecycle Methods in Action
Let's explore a practical scenario where these lifecycle methods come into play.
javascript
Copy code
import React, { Component } from 'react';
class LifecycleExample extends Component {
constructor(props) {
super(props);
this.state = {
data: [],
};
console.log('Constructor called');
}
static getDerivedStateFromProps(nextProps, nextState) {
console.log('getDerivedStateFromProps called', nextProps, nextState);
return null;
}
componentDidMount() {
console.log('componentDidMount called');
// Fetch data from an API
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => this.setState({ data }))
.catch(error => console.error('Error fetching data:', error));
}
shouldComponentUpdate(nextProps, nextState) {
console.log('shouldComponentUpdate called', nextProps, nextState);
// Example: Update only if the length of data changes
return nextState.data.length !== this.state.data.length;
}
getSnapshotBeforeUpdate(prevProps, prevState) {
console.log('getSnapshotBeforeUpdate called', prevProps, prevState);
return null;
}
componentDidUpdate(prevProps, prevState, snapshot) {
console.log('componentDidUpdate called', prevProps, prevState, snapshot);
}
componentWillUnmount() {
console.log('componentWillUnmount called');
// Cleanup operations, if any
}
render() {
console.log('render called');
return Rendered content;
}
}
export default LifecycleExample;
Conclusion
Understanding the React Native component lifecycle is crucial for developing efficient and performant applications. By strategically leveraging lifecycle methods, you can manage state, handle side effects, and optimize rendering. Whether you're initializing state in the constructor, fetching data in componentDidMount, or performing cleanup in componentWillUnmount, mastering the React Native component lifecycle empowers you to build robust and responsive mobile apps.
Add Comment
Web Design Articles
1. Why Choose A Website Development Company In Delhi?Author: Vikki kumar
2. Reactjs Vs Other Frontend Frameworks: Which One To Choose?
Author: Hetal V.
3. Top Reasons To Choose A Laravel Development Agency Over Freelancers
Author: Adarsh
4. Coindcx Clone Script: To Launch A Powerful Cryptocurrency Exchange Effortlessly
Author: Peterparker
5. Digital Marketing Mastery: Insights From San Diego Agencies
Author: Storm Brain
6. The Smart Way To Connect Ecommerce And Business Systems—powered By B2sell
Author: Gayathri
7. Top Website Design Chicago Companies To Watch In 2025
Author: Tim Harrison
8. The Future Of Ecommerce Development: Top Trends To Watch In 2025
Author: Manoj Singh
9. Lightspeed Squarespace Integration: The Concise Introduction
Author: Mary lindasy
10. Non-woven Industrial Membrane Market To Reach $2.22 Billion By 2030, Driven By Demand For Advanced Filtration Solutions
Author: Jackson smith
11. Salesforce Crm Consulting Services: Your Complete Guide To Smarter Customer Management
Author: Manoj Shrama
12. The 5 Best Programming Languages For Ai Development
Author: goodcoders
13. Pay Per Call Tracking Software
Author: Brian
14. What Trends In Ui/ux Design Await Us In 2025?
Author: Sanjay
15. Why Mobile-first Website Design Is Essential For Jaipur Businesses In 2025
Author: Aves Digital Agency